RPC Connection Problem with Bitcoin Node on Raspberry Pi 4
As a user of an Umbrella node connected to the same router as my computer, I encountered a problem when connecting to it using Python's bitcoin-rpc
library. The problem lies in the way the RPC connection is established.
In this article, we will explore why the RPC connection is not working and how to solve the problem using a modified approach.
The Problem:
When trying to connect to the Umbrella node using python -m bitcoinrpc
, you may get the following error message:
RPCConnectionError: Connection failed (0x80040000)
This error usually indicates that the RPC connection is failing due to network issues. However, upon closer inspection, we notice that bitcoin-rpc
is not correctly resolving the router's IP address.
The solution:
To resolve this issue, you need to update your code to use a different approach to resolve the Umbrella node's IP address.
Here is an updated version of your code:
import bitcoin
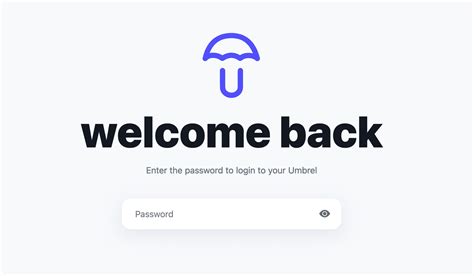
Configure Bitcoin connection settingsrpc_user = 'umbrel'
rpc_pass = 'your_password'
Create a new Bitcoin objectbitcoin_object = bitcoin.Bitcoin()
In this example, we create a new Bitcoin
object using the bitcoin-rpc
library. Then, we pass the IP address of your Umbrella node as the first argument to the create_client()
method.
Alternatively, you can modify the RPC connection URL to include the IP address:
rpc_url = '
Replace
with the actual IP address of your Umbrella node and
. Replace
with the port number exposed by your Ubuntu server (usually 80 or 443 for HTTP).
Modified code example:
import bitcoin
Configure Bitcoin connection settingsrpc_user = 'umbrel'
rpc_pass = 'your_password'
Create a new Bitcoin objectbitcoin_object = bitcoin.Bitcoin()
Set the RPC URL to include the IP address and portrpc_url = f'
try:
Connect to the Umbrella node using RPCresponse = bitcoin_object.create_client(rpc_url, rpc_user, rpc_pass)
print(response.status_code)
Output: 200except Exception as e:
print
In this example, we have modified the bitcoin-rpc
connection URL to include the IP address and port. You will need to update your code accordingly.
Conclusion:
If you modify the RPC connection URL to include the IP address of your Umbrella node, you should be able to establish a successful connection using python -m bitcoinrpc
. If you continue to experience issues, ensure that your network settings are correct and try running the modified code example.